On the Salesforce Ohana Slack, join link, someone asked “For a Flow: Does anyone know how I can extract the first letter of each word in a string?”. For example, if the string is “New York City”, the flow should output “NYC”. There was some discussion about this and using only the available Flow capabilities was said to be complex. This inquiring mind thought “How hard could this be?”. Here’s a Flow solution using native Flow capabilities.
See my Use Apex To Get First Letters In Each Word to see how to do this in Apex so one can compare and contrast the solutions.
Flow Solution Overview
An auto-launched flow, aka a sub-flow, is given an input string and it’ll output the first letter of each word into an output String that can then be used by the caller. If no characters found, an empty string is outputted.
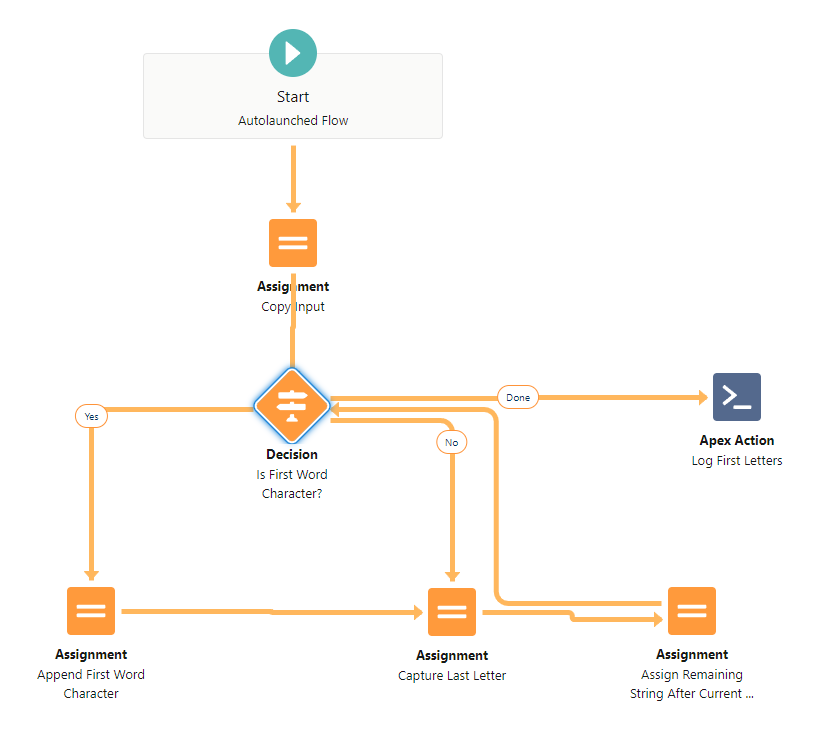
High-Level Algorithm
- Go through each character in the string one-by-one.
- If current character is a letter and the previous character is whitespace, append the current character to the list of first characters. When starting, the previous character is blank so this condition is handled too.
- Otherwise, advance to the next character.
- Repeat steps 2 and 3 until no more characters. If no words given, an empty string is outputted.
What’s tricky about flows is that their native text functions are limited to these according to here:
- BEGINS
- BR
- CASESAFEID
- CONTAINS
- FIND
- GETSESSIONID
- HTMLENCODE
- HYPERLINK
- IMAGE
- INCLUDES
- ISPICKVAL
- JSENCODE
- JSINHTMLENCODE
- LEFT
- LEN
- LOWER
- LPAD
- MID
- RIGHT
- RPAD
- SUBSTITUTE
- TEXT
- TRIM
- UPPER
- URLENCODE
- VALUE
Let’s now implement that algorithm in a Flow.
Create Autolaunched Flow
First, create an autolaunched flow named “Get First Letters of Each Word”. Next, let’s create the variables and formulas used in the various flow elements. Then let’s create the flow elements and try it out.
Create Variables
Create inputString Variable
First, create a text variable named inputString that allows one to pass in a string to the flow. The Default Value is an Empty String and it is “Available For Input”.
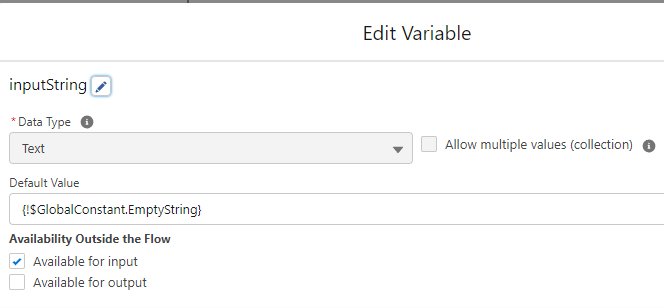
Create inputCopy Variable
Next, create a text variable named inputCopy that is a copy of the input string. The Default Value is an Empty String and isn’t available outside the flow. This technically isn’t needed but lets one have a copy to manipulate the string as needed.
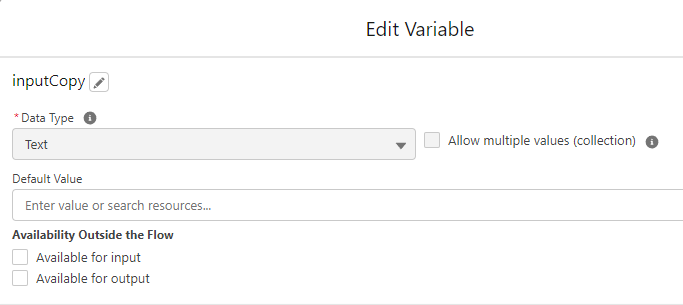
Create firstLetters Variable
Next, create a text variable named firstLetters as an output to hold the first letter of each work in the inputString. The Default Value is an Empty String and is “Available for Output” outside the flow.
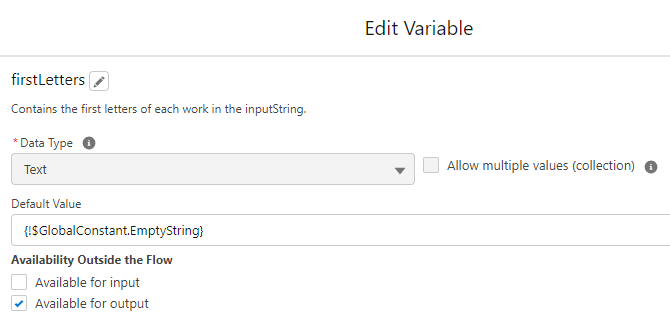
Create lastLetter Variable
Next, create a text variable named lastLetter to hold the previous letter right before the current one. The Default Value is an Empty String and is not available outside the flow.
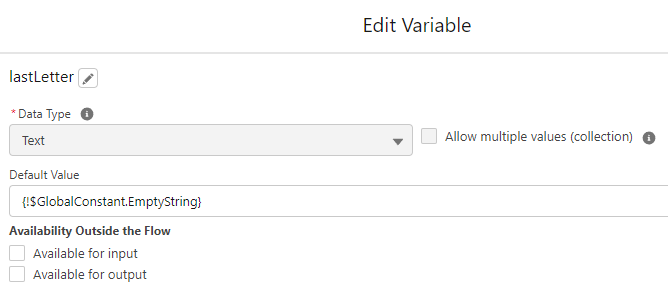
Create Formulas
Next, create the formula fields used to parse the string into different parts. Each is used in the main flow logic to implement the algorithm.
Create currentLetter Formula
Create a formula named “currentLetter” with a Text data type. It’s Formula body is:
IF( IsBlank({!inputCopy}) = False,
LEFT({!inputCopy}, 1),
'')
This formula is used to fetch the first letter in the inputCopy. The inputCopy has its first character removed after each iteration so this formula is used to fetch the currentLetter at the beginning. If the inputCopy is blank, then the empty string is returned. Otherwise, first character is returned by using Left(inputCopy, 1).
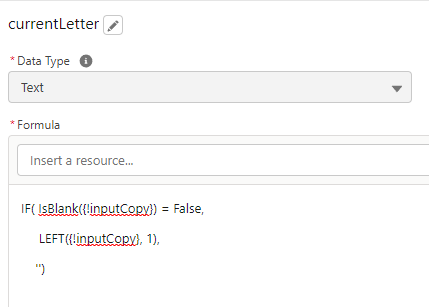
Create currentLetterIsNotWhiteSpace Formula
Next, create the currentLetterIsNotWhiteSpace formula with a Boolean data type. This is useful to determine if the current letter is whitespace such as a space, tab or a newline or not. Formula Body:
IsBlank( Trim( {!currentLetter} )) = False
This code says first trim the currentLetter which removes any whitespace at the beginning and end of the string given and returns the result. The trimmed current letter is then passed to IsBlank to see if it’s an empty string or not. If false, the currentLetter is not white space. It true, it is whitespace.
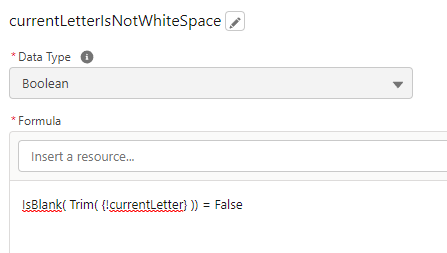
Create lastLetterIsBlankORWhiteSpace Formula
Create the “lastLetterIsBlankORWhiteSpace” formula with a Boolean data type. This formula determines if the previous letter, the one before the current one, is white space or not. Formula body:
ISBlank( TRIM( {!lastLetter} ) )
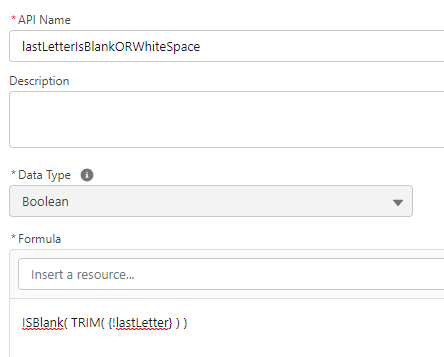
Create remainingStringAfterFirstLetter Formula
Now, create the “remainingStringAfterFirstLetter” formula with a Text data type. This formula is used to gather the rest of the string after the current letter. It’s used to update the inputCopy with the remaining string after the current string to essentially advance the current letter in the main loop.
Formula Body:
IF (LEN ({!inputCopy}) > 1,
RIGHT({!inputCopy}, LEN ({!inputCopy}) -1 ),
"")
If the length of the current string is 2 or more characters, then take the right most length – 1 characters omitting the first character. Otherwise, return the empty string if there’s 0 or 1 characters.
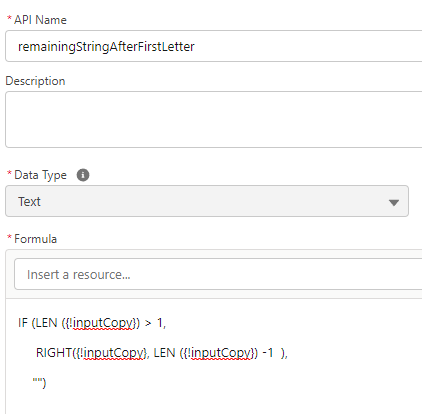
Create Flow Elements
First, change from the auto-layout to the Freeform layout so one can create a “while” loop via a decision element and its elements that follow.
Create Copy Input Assignment
First let’s create a copy of the inputString so the string can be manipulated as needed without disturbing the original value. This is done by assigning the inputString value to the inputCopy value in the Copy Input assignment. Connect the start element to this new assignment element.
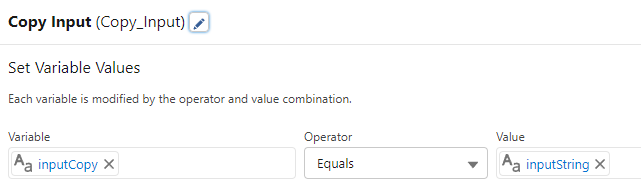
Create “Is First Word Character?” Decision
Next, create the “Is First Word Character?” decision.

This decision has 3 outcomes:
- Done
- Yes
- No – This is the default
Create the outcomes using the screen shots below.
Done Outcome
When the current letter is blank or null, then stop since the whole string has been traversed.
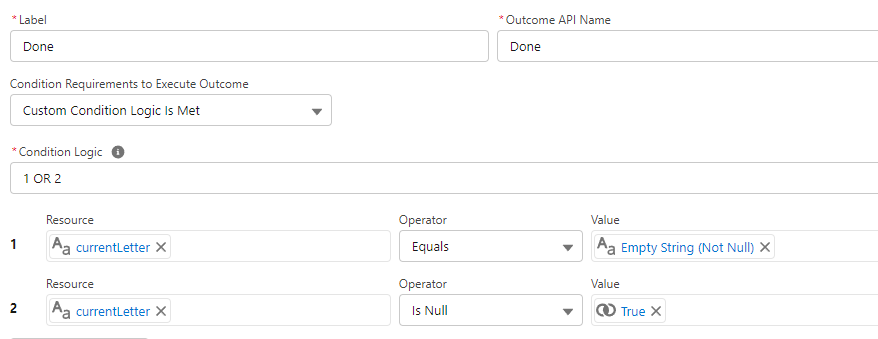
Yes Outcome
When the current letter is not empty and not white space and the last letter is blank OR whitespace, then you know that the current letter is the first letter in a word. This works for the first word since the lastLetter is initially set to an empty string.
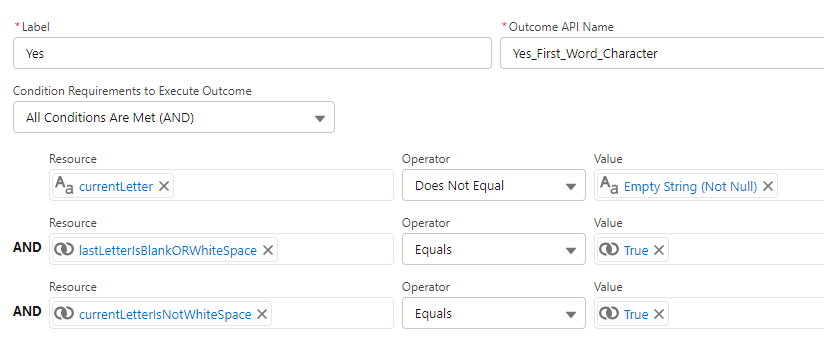
No Outcome
Rename the Default Outcome to No.
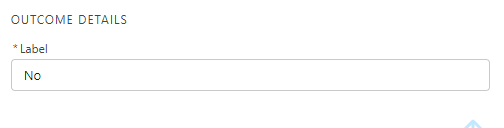
Connect the “Copy Input” assignment element to this decision.
Create “Append First Word Character” Assignment
Next, create the “Append First Word Character” assignment to append the “currentLetter” to the firstLetters variable using the following screen shot.
Connect the “Is First Word Character?” decision to this assignment using the “Yes” outcome.
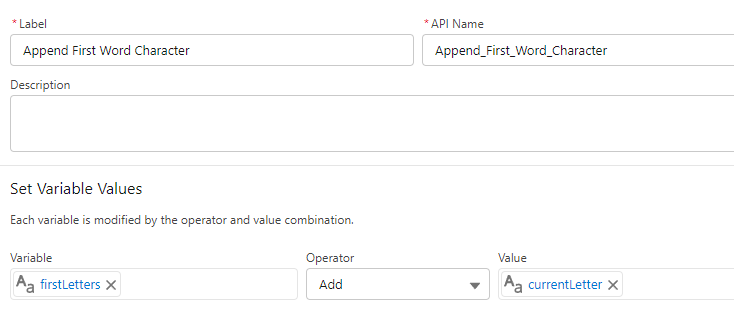
Create “Capture Last Letter” Assignment
Next, create the “Capture Last Letter” assignment to assign the currentLetter to the lastLetter variable so it can be used in the next iteration. Use the screen shot to create the assignment.
Next, connect the “Append First Word Character” element to this assignment. Then connect the “Is First Word Character?” decision to this assignment using the “No” outcome. After the First word’s letter is appended or it’s not the first character, we want to capture the last letter and move on.
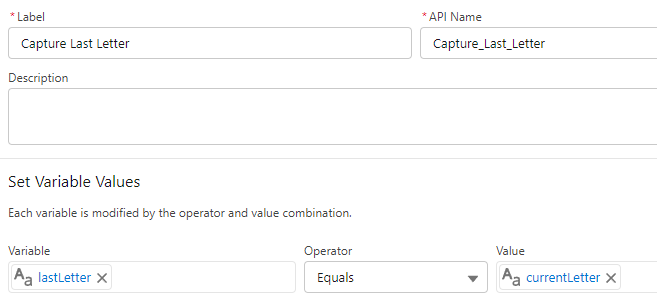
Create “Assign Remaining String After Current Letter” Assignment
Next, create the “Assign Remaining String After Current Letter” assignment that sets the inputCopy variable to the remaining characters in the string after the current character aka current letter using the “remainingStringAfterFirstLetter” formula to fetch the remaining characters. Use the screen shot for details.
Now connect the “Capture Last Letter” to this assignment. Next, connect this assignment back to the “Is First Word Character?” decision to complete the loop.
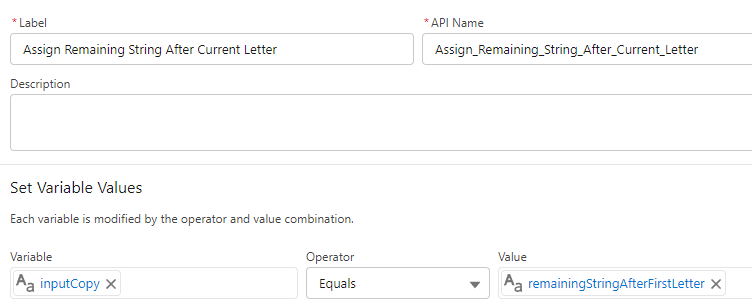
After completing the create flow element steps, the flow should look something like this:
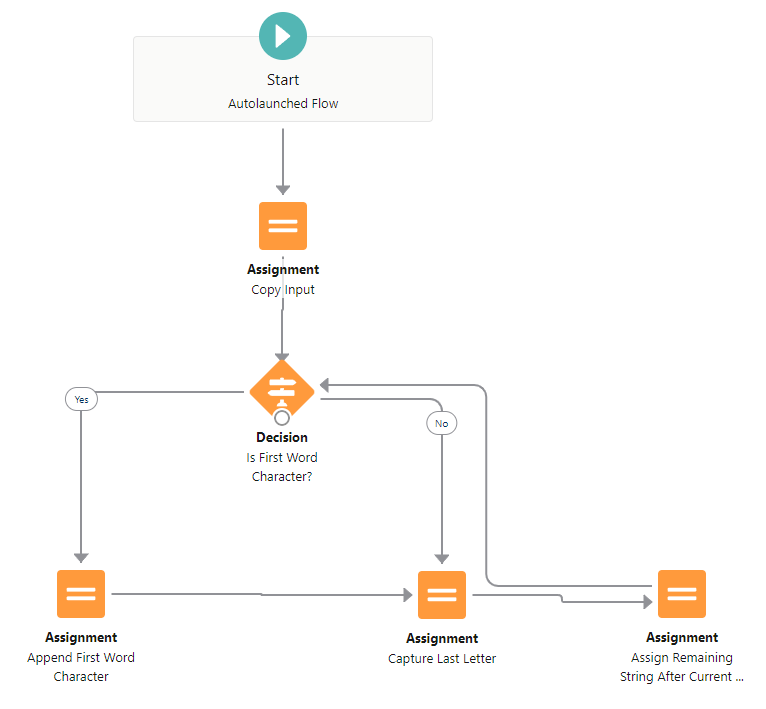
Save the flow so no work is lost.
Activate it when ready to use and use it in other flows as needed keeping in mind the considerations below.
Testing it Out
To test it out, one can use the Debug button but one has to output the “firstLetters” variable somewhere so it’ll show up in the debug details. Otherwise, it’s value is hidden. Using my Message Logger Action, one can do that easily by adding a new “Log First Letters” action and connecting the Done outcome from the “Is First Word Character?” decision to it like this:
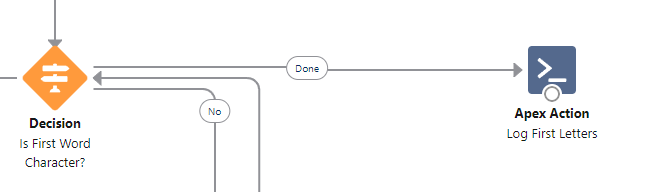
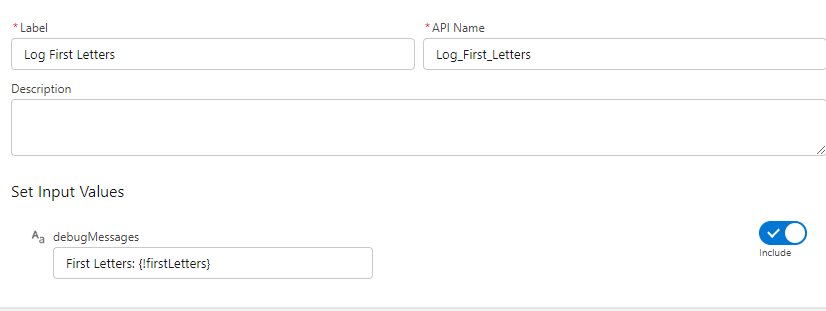
Now when the Debug button is clicked, enter a value in “inputString” and click the Run button. For example, enter “New York City” and the firstLetters should be “NYC”. At the bottom of the log, expand the Log Action and you should see …. “First Letters: NYC” like this:
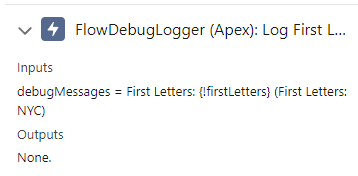
Considerations
- Not Scalable. Since flows are limited to 2,000 elements being executed in a flow interview, this is limited to at most 600-700 character strings which is not very long. A better solution is to invoke some Apex to do the heavy lifting and then return the results since Apex has better string functions and that will allow much longer strings.
- This was done to see if this was possible and to show others how it could be done.
- There are probably better algorithms but this was the easiest that came to mind.
What did you think?