Yes that’s a thought provoking title! Let’s see why… But first a definition…
Programming – The instructions given to a computer or a computer system to fulfill a task. If you expand the definition on dictionary.com, #11 has the same definition as mine but mine constrains it to computers.
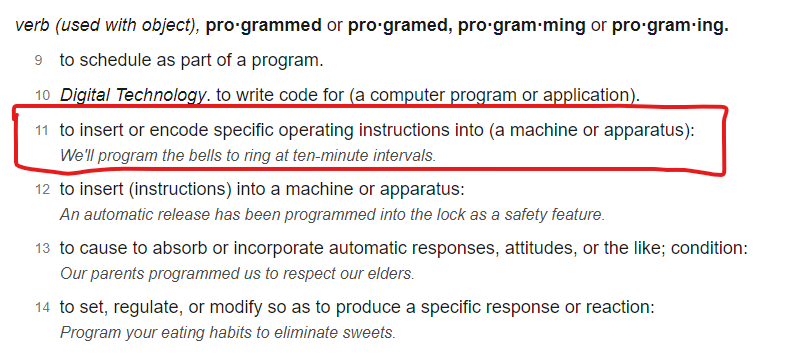
Why?
Salesforce and many others in the ecosystem market flows as “Low or no code”. However, my take is that flows are simply another way of programming besides using Code. If you can learn Flows, you can learn to write code too because they use the same fundamental building blocks! Those are sequencing, selection and iteration!
Sequencing
Sequencing is the order in which the instructions, sometimes called operations, are executed. In Flows, this starts with the start element and follows the arrows to the next element aka instruction. In Apex, and many other programming languages, the instructions run top-to-bottom.
Let’s take a look at an example of doing the same task using a flow and then in Apex. The task is to assign two numbers to two different variables, adding them together, and then storing the result in a third variable.
Flow Sequencing Example
For brevity sake, I’m omitting declaring the variables in the flow. First, let’s assign the number 0 to the variable i.
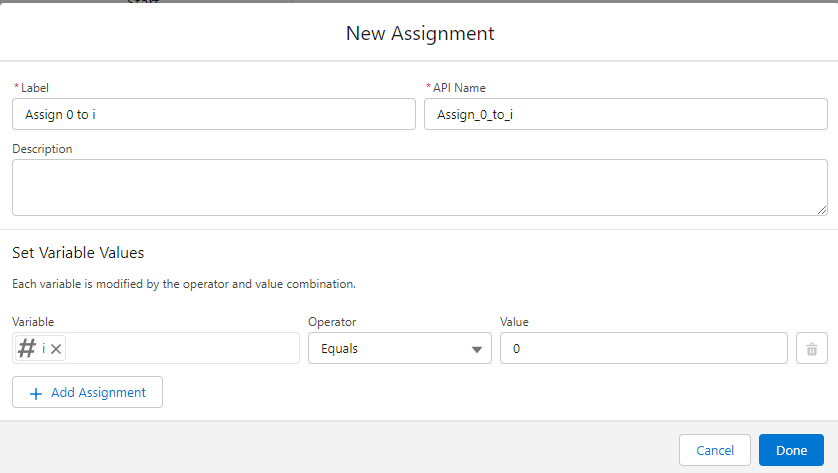
Next, let’s assign the value 1 to the variable j.
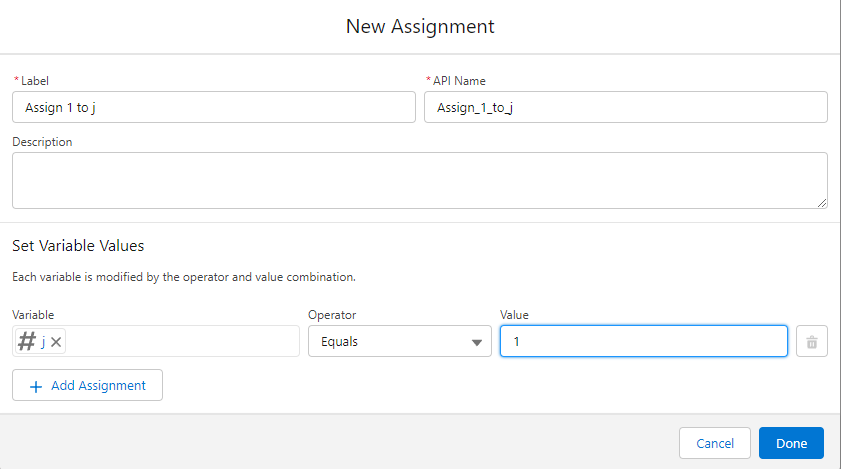
Now, let’s add the variables i and j together using a formula since one can’t do that in an assignment element directly!
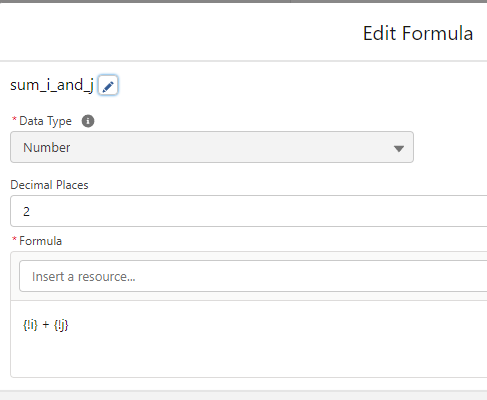
Now, the sum, from the formula, can be used to add i and j together and store the result in the sum variable.
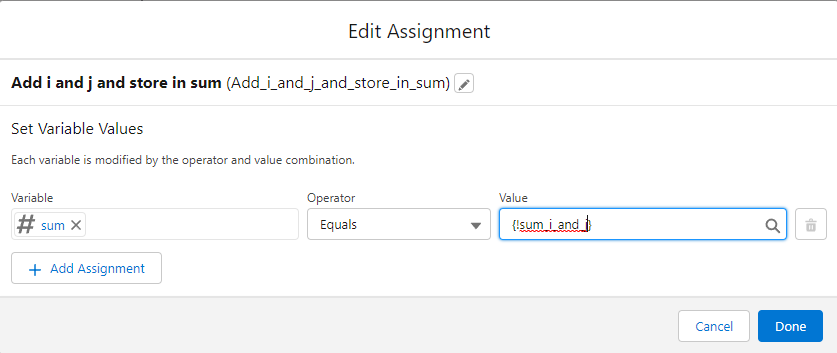
Here’s what the instructions look like on the canvas:
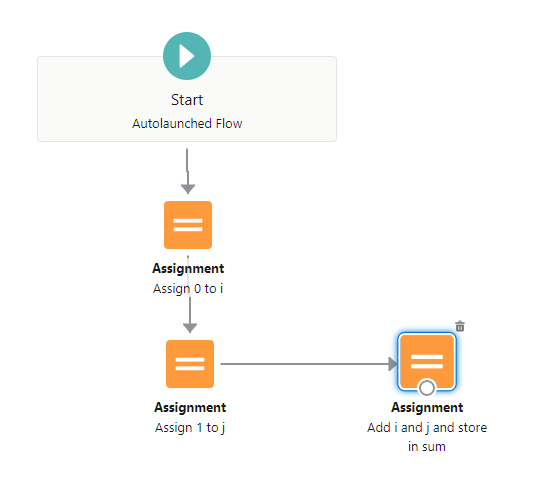
Apex Sequencing Example
Integer i = 0; // The variable i is declared and the number 0 assigned to it.
Integer j = 1; // The variable j is declared and the number 1 assigned to it.
Integer sum; // The variable sum is declared without any value assigned.
sum = i + j; // i and j are added together and the result assigned to sum.
Selection
Selection are instructions to run different sets of instructions depending on some condition. In Flows these are decision elements. In Apex, these are “if” and “case” statements.
Let’s expand on our previous example to do something if the sum is greater than 10 and do something else if the sum is 10 or lower.
Flow Selection Example
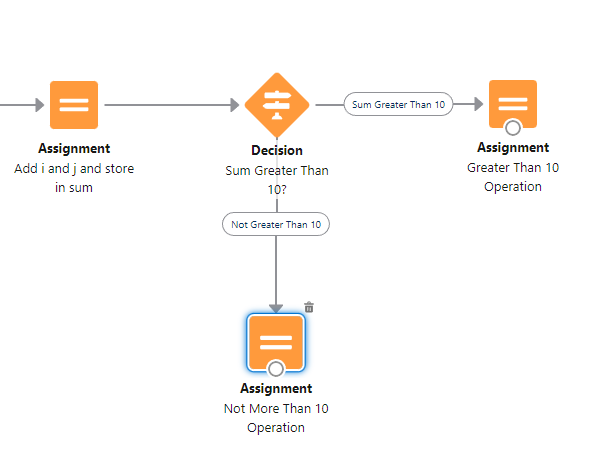
Apex Selection Example
// ... Other code omitted for brevity
sum = i + j; // i and j are added together and the result assigned to sum.
if (sum > 10) {
// sum is greater than 10 so do something here
}
else {
// sum is NOT greater than 10 so do something else here
}
Iteration
Iteration are the instructions that let one repeat a set of instructions in different ways. For example, you want to do a set of instructions for a given number of times. Another example is one has to go through all the items in a collection, such as a list or an array, and do something with each item.
In Flows, one can use the “Loop” element along with other elements to do this. One can also build a loop using a decision element and other elements that circle back to the decision element. That can be used to build a traditional “for” loop or “while” loop.
In Apex, there are traditional for loops, while loops, and for each loops.
Let’s look at an example using a for each loop in Flows and Apex where we want to filter all accounts whose annual revenue is greater than $1,000,000 into the VIP accounts collection variable. The for each is used because the “loop” element in flows corresponds to the for each construct in Apex.
Iteration Flow Example
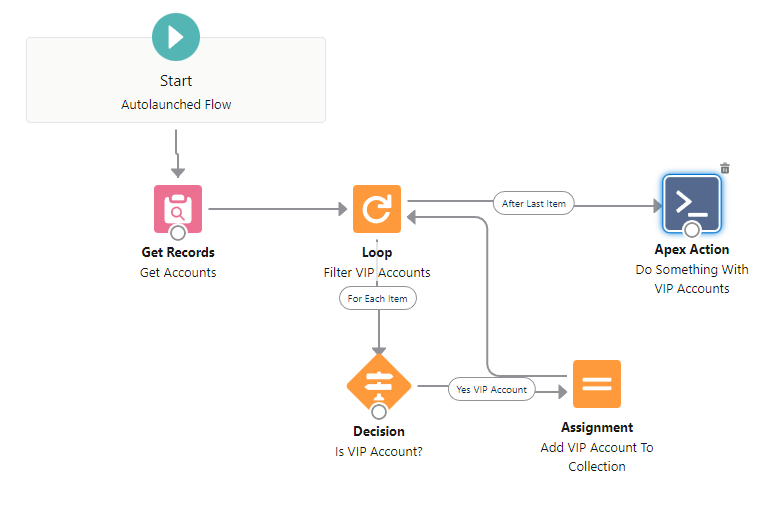
Get Accounts Definition
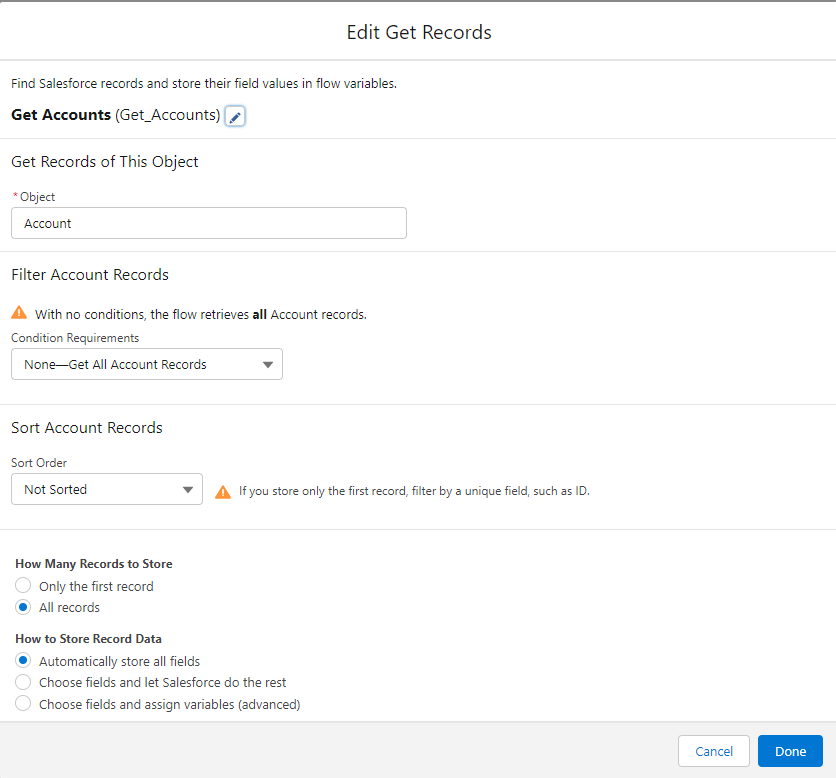
Filter VIP Accounts Loop Definition
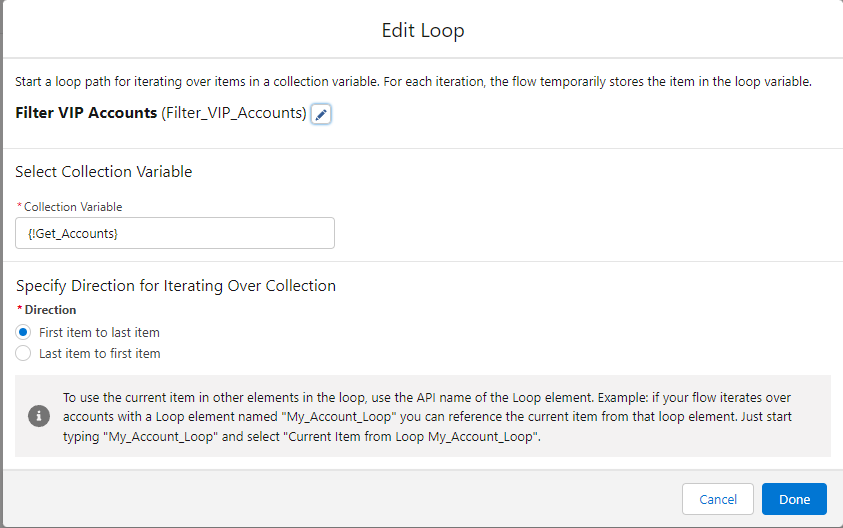
Is VIP Account Decision Definition
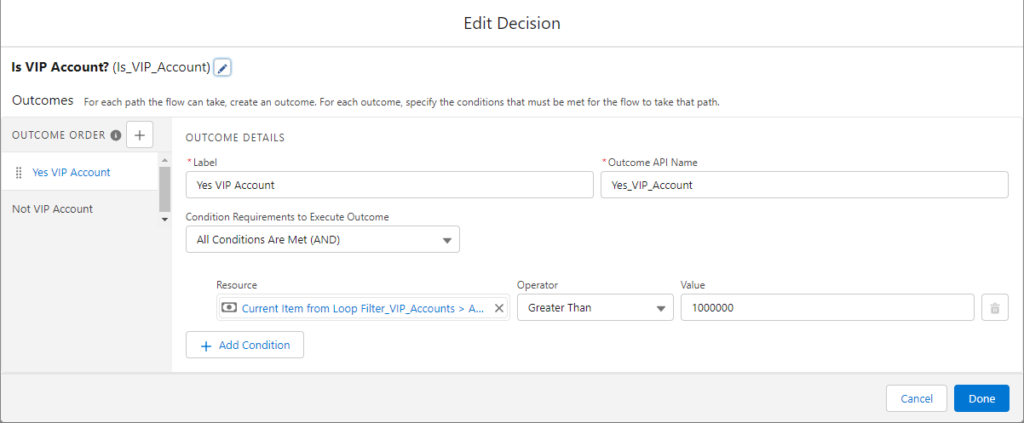
Add VIP Account To Collection Definition
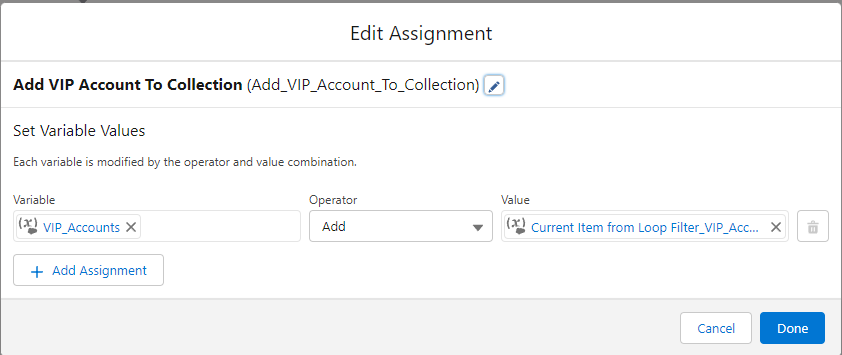
Iteration Apex Example
List<Accounts> eligibleVIPAccounts =
[SELECT Id,
AnnualRevenue
FROM Account];
List<Account> vipAccounts = new List<Account>();
for (Account eligibleVIPAccount : eligibleVIPAccounts) {
if (eligibleVIPAccount.AnnualRevenue > 1000000) {
vipAccounts.add(eligibleVIPAccount);
}
}
// vipAccounts now has any found VIP accounts so do something with them.
Note: One can use a SOQL query to filter the accounts directly for this particular example but I wanted to show a direct comparison to Flows.
Back in my Programming Languages class at university, we were taught that sequencing, selection and iteration can be combined to create any program. Also, when you find out how a programming language does sequencing, selection, and iteration, you now know the fundamentals of that programming language. Since Flows have these three capabilities, my contention is that it’s a programming language albeit a “graphical” one.
Since flows are a programming language, a flow builder is a programmer. If a flow builder is comfortable with building flows, then learning Apex code will probably be easy to learn the fundamentals. Learning Object Oriented programming, recursion and other Apex features can come later.
So dear reader, did I convince you? If not, let me know in a comment.